Public Transport Mobility Simulation
Abstract
This project models and simulates the interactions between passengers, public transport vehicles (buses), roads, and bus stops in a dynamically evolving urban environment. Built using Mesa, a Python-based multi-agent simulation framework, it evaluates the performance and resilience of public transport systems under normal operations and during disturbances such as road failures or vehicle breakdowns.
📹 Demo Video
—
Problem Definition
Objectives
- Passenger and Vehicle Simulation:
- Represent passenger behaviors, including waiting at bus stops and traveling by bus.
- Model buses with predefined routes and schedules, adapting to real-time conditions.
- Impact of Disturbances:
- Introduce road disconnections.
- Quantify their effects on passenger travel times and system efficiency.
- System Resilience:
- Develop adaptive strategies to mitigate disruptions in transport systems.
Design and Implementation
Architecture
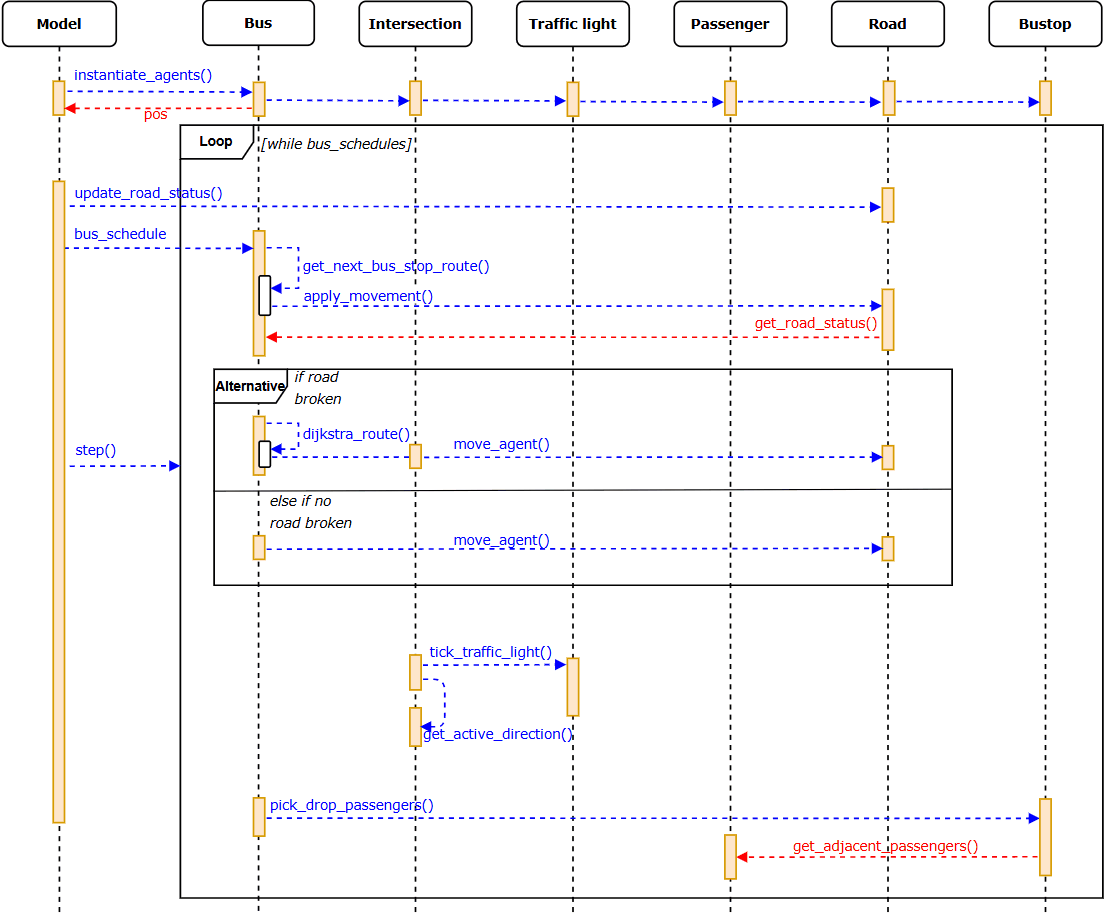
Design Process
- System Breakdown:
- Modularized components such as roads, buses, and passengers for clarity.
- Agent Interactions:
- Designed interactions like bus-passenger pickups, road conditions affecting movement, and dynamic rerouting.
- Disturbance Management:
- Incorporated real-time road failures and recovery mechanisms to analyze their impacts.
Coding Practices
- Object-Oriented Design:
- Encapsulated agent behaviors into dedicated classes for clarity and reuse.
- Modular Codebase:
- Organized the system into separate Python modules for roads, buses, passengers, and more.
- Documentation:
- Used concise docstrings and Sphinx for automated documentation.
System Components
Environment
The city is represented as a 2D grid containing:
- Roads: Routes for bus movement, marked operational or broken.
- Bus Stops: Locations where buses pick up or drop off passengers.
- Intersections: Nodes where roads meet, managed by traffic lights.
- Traffic Lights: Control allowed directions for bus movement.
- Sidewalks: Paths passengers use by foot, which buses cannot traverse.
Agents
Passenger
- Attributes:
pos
: Current position in the grid.
destination
: Target position for the passenger.
is_traveling
: Indicates if the passenger is on a bus.
is_waiting
: Indicates if the passenger is waiting for a bus.
has_arrived
: Indicates if the passenger has reached their destination.
- Behaviors:
- Request rides from buses at bus stops.
- Board buses if space is available.
Bus
- Attributes:
busSchedule
: Predefined schedule detailing stops and routes.
name
: Unique identifier for the bus.
capacity
: Maximum number of passengers it can carry.
pos
: Current position in the grid.
destination
: Final destination of the bus.
direction
: Current movement direction.
route
: Directions to the next bus stop.
passengers
: List of passengers currently on board.
- Behaviors:
- Follow schedules, stopping at designated bus stops.
- Pick up or drop off passengers based on destinations.
- Dynamically reroute when encountering road failures.
Bus Stop
- Attributes:
pos
: Location of the bus stop.
waiting_passengers
: List of passengers waiting for a bus.
- Behaviors:
- Serve as a meeting point for buses and passengers.
- Manage waiting passengers.
Road
- Attributes:
status
: Indicates if the road is operational or broken.
- Behaviors:
- Toggle between operational and broken states due to disturbances.
- Block buses from traveling on broken roads.
Intersection
- Attributes:
traffic_lights
: Manage traffic direction at intersections.
directions_to_go
: Allowed traffic directions.
pos
: Position on the grid.
active_light
: Currently active traffic light.
ticks_to_light_change
: Time until the next light change.
- Behaviors:
- Rotate traffic lights to alternate directions.
Traffic Light
- Attributes:
status
: Current state (red, yellow, green).
direction
: Direction controlled by the light.
- Behaviors:
- Regulate bus movement based on light status.
- Transition between green, yellow, and red.
Challenges and Solutions
Challenges
- Dynamic Rerouting:
- Problem: Calculating efficient routes during road failures.
- Solution: Integrated Dijkstra’s algorithm for real-time pathfinding.
- Synchronization of Agents:
- Problem: Ensuring smooth interactions between buses, passengers, and roads.
- Solution: Leveraged Mesa’s staged activation to execute agent behaviors sequentially.
- Scalability:
- Problem: Maintaining performance with increased grid size and agents.
- Solution: Optimized agent interactions and ensured modular design for scalability.
Results and Observations
Key Metrics
- Passenger Travel Time:
- Measured time taken from origin to destination under normal and disturbed conditions.
- Bus Efficiency:
- Evaluated by the number of passengers served per route.
- Impact of Disturbances:
- Quantified delays caused by road failures and breakdowns.
Insights
- Resilience:
- Adaptive rerouting significantly improved system robustness against disturbances.
- Traffic Management:
- Optimized traffic light operations reduced congestion.
Future Enhancements
- Dynamic Passenger Behavior:
- Simulate passengers choosing alternative routes or modes of transport.
- Advanced Traffic Control:
- Utilize machine learning for predictive traffic light scheduling.
- Expanded Transportation Modes:
- Include additional vehicles like taxis or bicycles for richer simulations.
Getting Started
This guide provides two ways to set up and run the simulation:
- Using Conda Environment
- Using Docker Hub
1. Running with Conda Environment
Prerequisites
- Python 3.10+
- Conda (Miniconda or Anaconda)
Step-by-Step Guide
- Clone the Repository:
git clone https://github.com/CongSon01/UGE-MAS_BusSimulation
cd UGE-MAS_BusSimulation
- Create a Conda Environment:
conda create --name bus_simulation python=3.10 -y
conda activate bus_simulation
- Install Dependencies:
pip install -r requirements.txt
- Run the Simulation:
2. Running with Docker Hub
- Pull the Pre-Built Docker Image:
docker pull congson01/mobility-simulation:latest
- Run the Docker Container:
docker run -p 8521:8521 congson01/mobility-simulation:latest
3 Access the Simulation: